Table of Contents
GPIO Theory
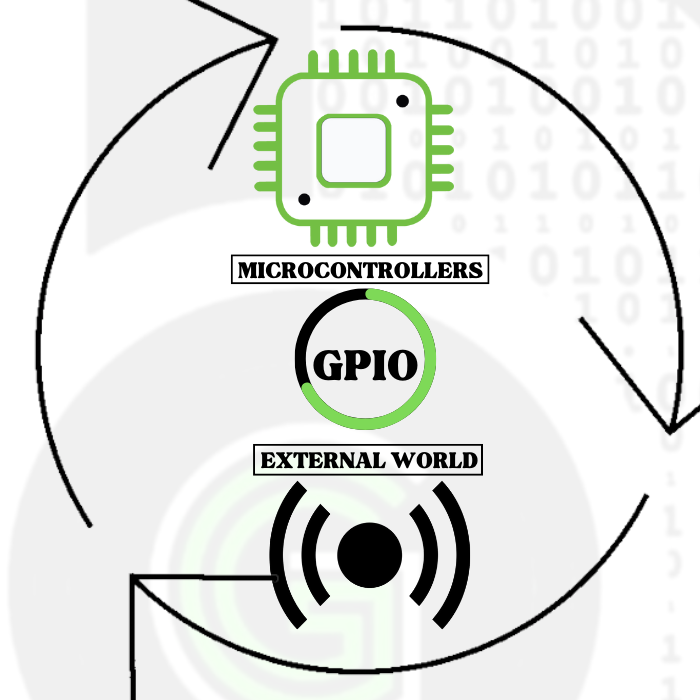
So now we’ll talk about GPIO pins . GPIO stands for general purpose input output pins; they are the means for communication between the microcontroller and the external world (using sensors etc).It is signal that arrives on these pins or a signal that is sent or written on these pins that facilitates this communication. These pins can be configured to act as input or output via the application software(in our case stm32cube ide). The programmer can configure the pins as LOW or 0V or HIGH or 3.3V(or 5V ).
These GPIO pins can also be configured to act as special purpose pins as well where their alternate functionality is exploited . These alternate functionality includes UART , ADC , SPI etc . In the case of UART communication, Transmitter(Tx) and Receiver(Rx) pins are required. GPIO pins can be configured to act as TX or RX pins . Even in ADC the pins are configured to act as Analog pins having 12 bits of resolution . The alternate functions of various pins are shown below:
Input floating
Input pull-up
Input pull-down
Analog
Output open-drain
Output push-pull
Alternate function push-pull
Alternate function open-drain
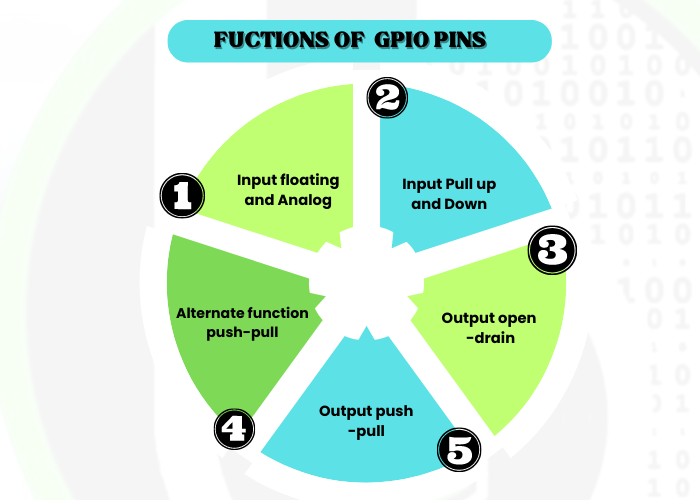
GPIO Peripheral in STM32F103
All the pins of STM32F103 are grouped in multiple ports as PORT A, PORT B, PORT C As can be seen from Pin configuration chart in the PA1 stands for Port A Pin 1. There are 37 GPIO pins in stm32f103 which are divided as PORT A with 16 pins, PORT B with 16 pins, PORT C with 3 pins and PORT D with 2 pins.
- Each GPIO port has two 32-bit configuration registers (GPIOx_CRL, GPIOx_CRH), two 32-bit data registers (GPIOx_IDR, GPIOx_ODR), a 32-bit set/reset register (GPIOx_BSRR), a 16-bit reset register (GPIOx_BRR) and a 32-bit locking register (GPIOx_LCKR).
- In the register names, x stands for the port to which pin belongs. If we are configuring pin PA1, it has Port A then registers would be accessed by GPIOA_CRL and etc.
- Out of above-mentioned registers, GPIO peripheral has 2 most important registers:
GPIO Peripheral Block Diagram in STM32F103
- First after selecting the pin the port is decided
- Then after following the arrow the busses are selected based on it: APB/APB1 or AHB
- After which the clock is enabled to the particular port using either __HAL_RCC_GPIOX_CLK_ENABLE() function or using the RCC AHB1 peripheral clock enable register and selecting the port to which clock has to be provided by enabling it.
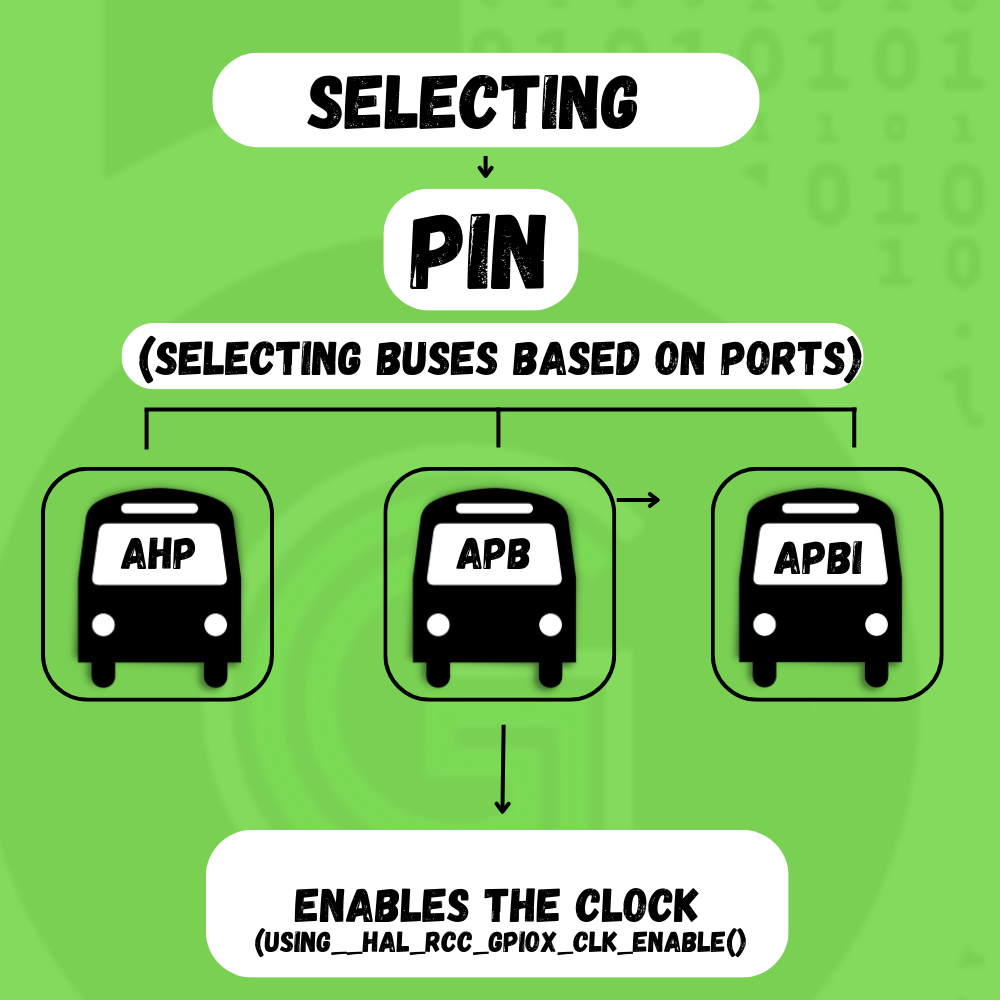
Methods to configure the GPIO Peripheral
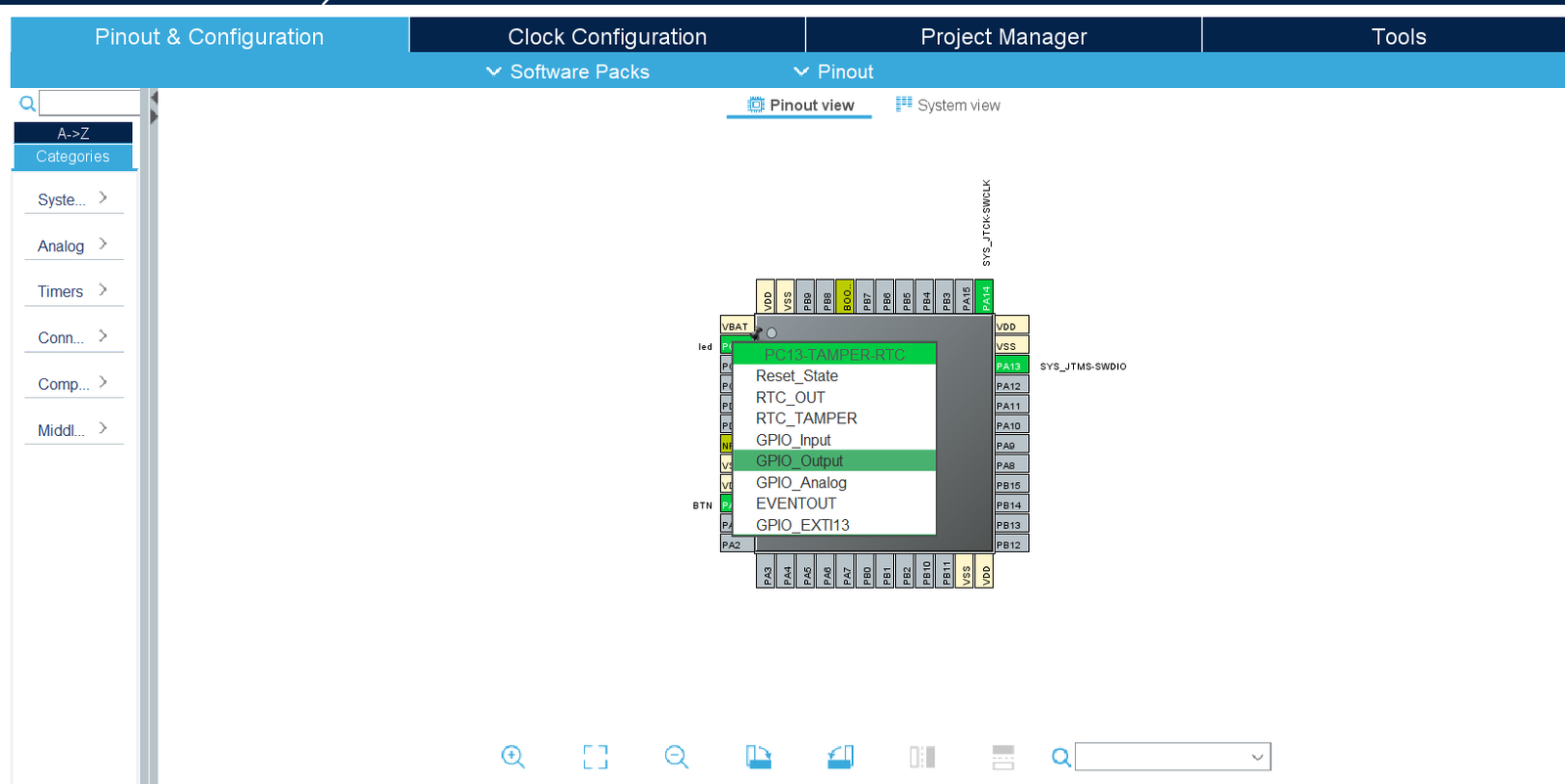
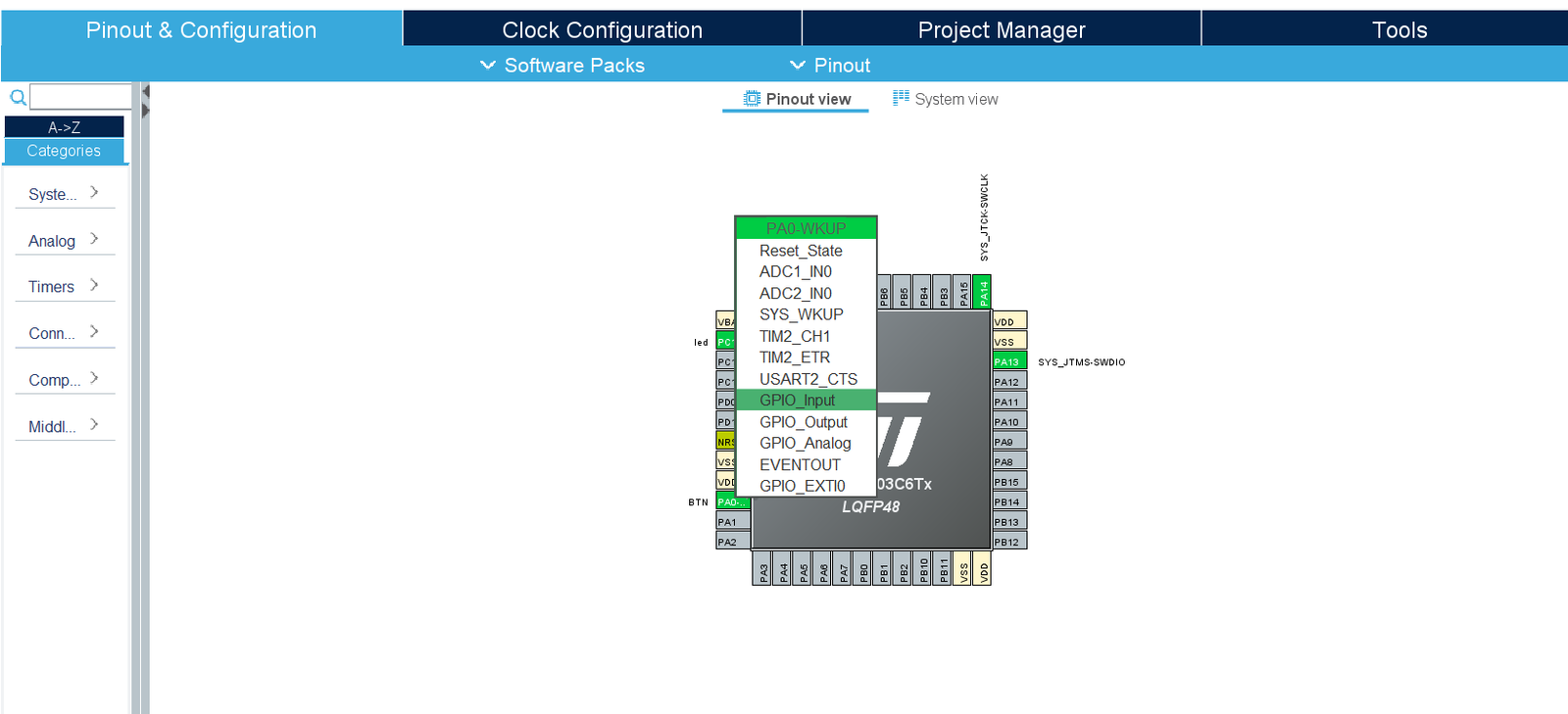
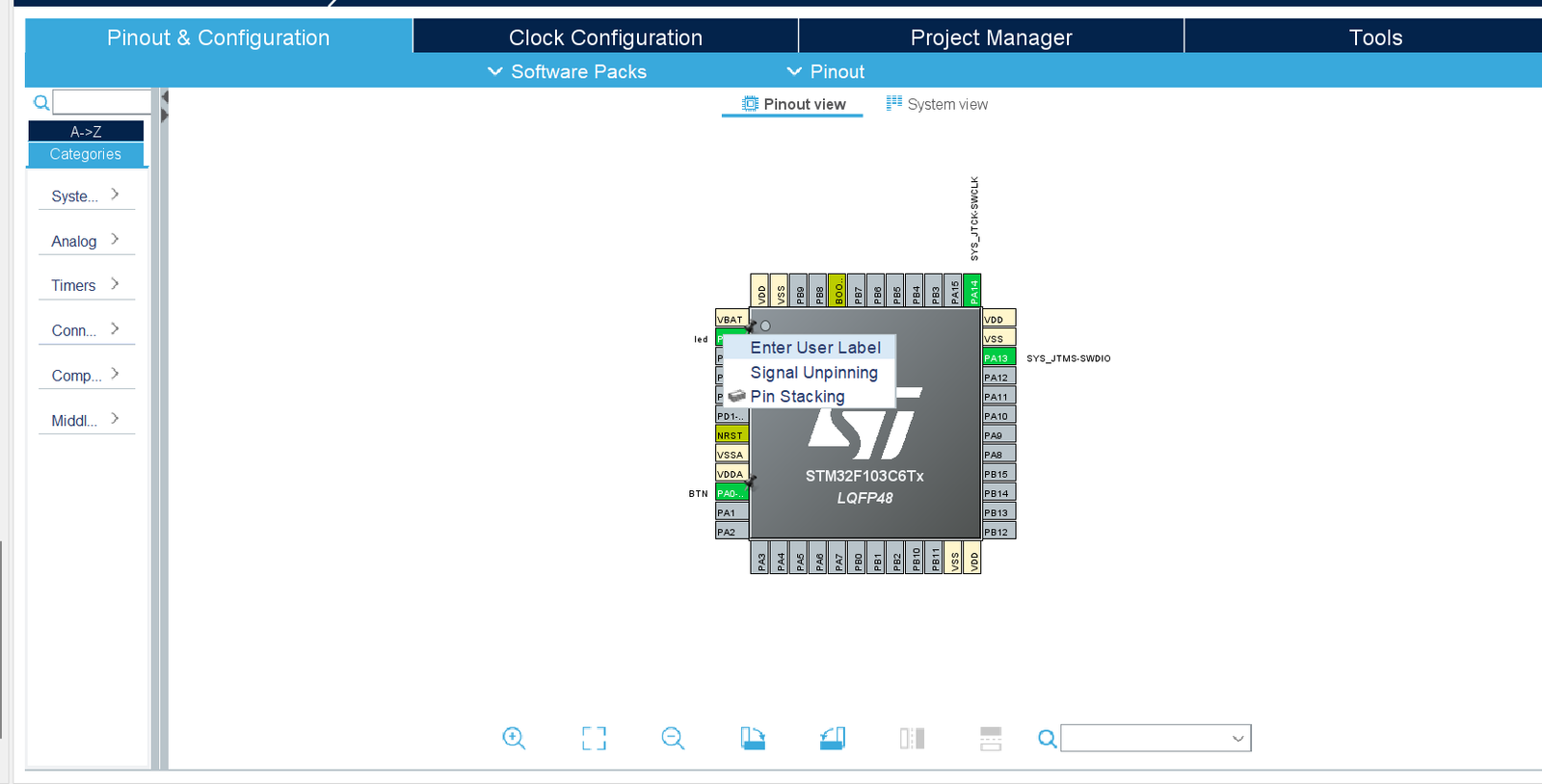
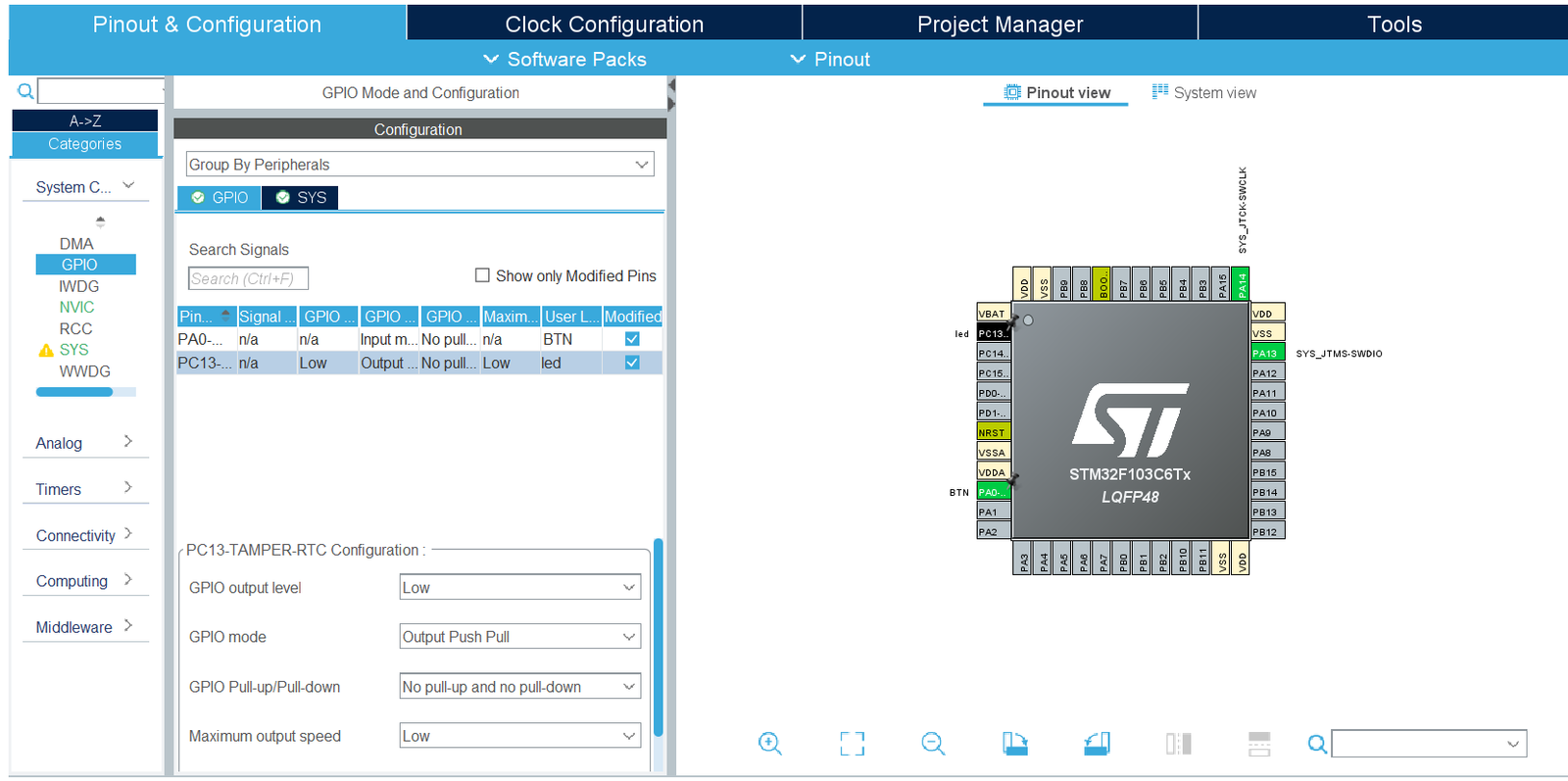
- Configuring the busses i.e AHB1, AHB2, APB1, APB2 . The AHB bus is faster than APB bus and in case of certain modules they are connected to the same bus .Hence it depends upon the application which bus to use. As can be seen from the picture below the AHB1 takes clock to PORT A , PORT B , PORT C etc . Hence to initialize a pin to a particular port the in RCC AHB1 clock enable register GPIOEN is set to 1 (For Port A GPIOAEN , For Port B GPIOBEN etc)
- Enabling the clock to that port otherwise the particular pin will not be functional
- Creating an instance of the structure and then using the members of the structure set the following:-
- PIN – Takes the pin no as input GPIO_PIN_X {where X -0 to 15}
- MODE– Selects the mode the specified pin is supposed to work in . It takes in value Output Push Pull ,Output Open drain
- PULL- It selects the initial value of the pin and takes value no pull up no pull down, pull up or pull down
- SPEED- Selects the speed of the working of the specified pin i.e low, medium or high
- ALTERNATE- Specifies the alternate function performed by the pin UART TX OR RX , ADC etc,
GPIO Peripheral SDK using STM32HAL
We are going to use STM32 HAL SDK for using the GPIO peripheral of the STM32F103. STM32HAL is a very versatile and robust Software package for using Peripherals of the STM32 Microcontroller family. To know more about STM32HAL, refer to this link.
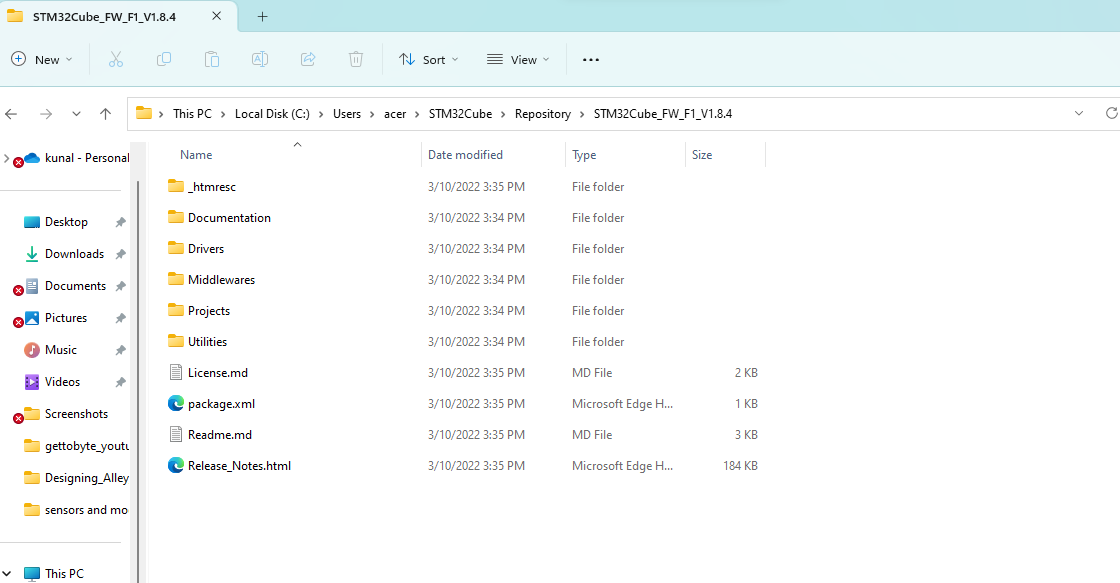
Each STM32 HAL has drivers for all the peripherals of the STM32 Microcontroller(One can navigate to the Driver folder in the STM32F1 HAL local repo installed). These drivers can be configured and enabled to use in the project via the STM32 CubeMX configuration tool, which is also integrated into STM32CubeIDE( just like told in the above section for configuring GPIO peripherals). Will be digging into that part, in the next section. For now, let’s understand the STM32HAL GPIO SDK for STM32F103 MCU.
- stm32f1xx_hal_gpio.c: This file consists of various macros and is responsible for the intialization and configuration of the functions which in turn configures the peripheral.
- stm32f1xx_hal_gpio.h: consists of various structure definitions that help configure various parameters of the pin, enumeration, and various macros
- stm32f1xx_ll_gpio.c & stm32f1xx_ll_gpio.h: GPIO Low-level driver source/header file, contains functions that configure the GPIO Peripheral registers at the hardware level. These files are the ones that actually interact with the hardware and make it configurable to our needs. 
STM32 HAL Functions for GPIO Peripheral
Functions are set of instructions that required to perform certain tasks. In general, a function is first declared in header file(.h) and then it is definied in source file(.c) and then called in main.c or application code.
It is of the form function return data type, function name and function arguments.
// Basic introduction of functions in c
void func(int x);
int main()
{
func(x);
}
void func(int x)
{/*function body/*}
In Embedded functions are required to initialize a peripheral or configure it on the basis of various parameters which are passed on using arguments. This information is then passed on to the registers.
List of functions used for GPIO HAL:
FUNCTION NAME
HAL_RCC_GPIOX_CLK_ENABLE()
FUNCTION DESCRIPTION
This API is used to enable the clock to a particular port that will be delivered using AHB OR APB busses. This function basically sets the APBENR bit in RCC register and after a delay enables the clock.
RETURN VALUE
NONE
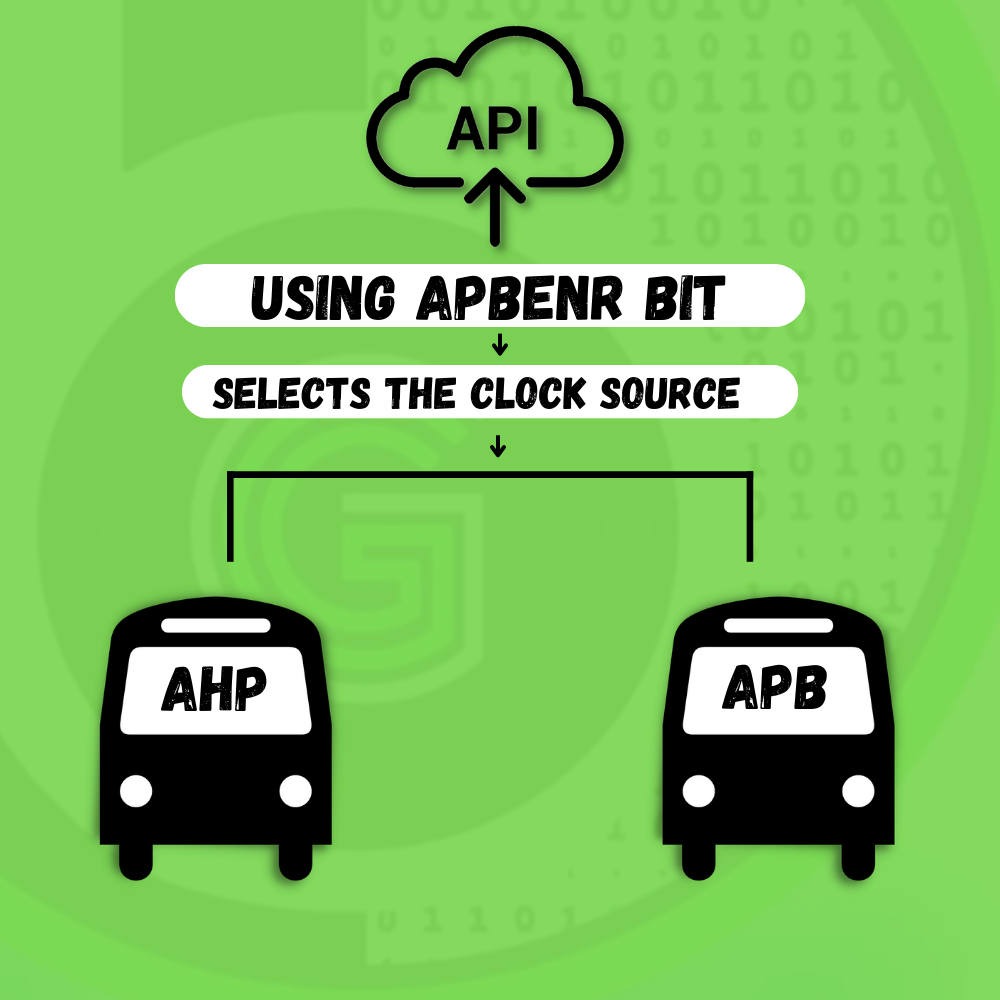
USAGE
static void MX_GPIO_Init(void)
{
/* GPIO Ports Clock Enable */
__HAL_RCC_GPIOC_CLK_ENABLE();
}
FUNCTION NAME
- void HAL_GPIO_Init (GPIO_TypeDef* GPIOx, GPIO_InitTypeDef *GPIO_Init)
FUNCTION DESCRIPTION
This API takes 2 arguments as input that are pointer to structure GPIO_TypeDef* GPIOx this is usually used to specify the port (A,B etc) and GPIO_InitTypeDef *GPIO_Init which is basically the pointer to the structure GPIO_InitTypeDef this contains information of the pin configuration such as pin , mode , speed , pull , alternate. This API basically initializes the specified pin according to configuration specified in GPIO_Init.
PARAMETERS (ARGUMENTS)
GPIOx:specifies the PORT ie X(A,B,C)
GPIO_Init: Pointer to GPIO_InitTypeDef that has the configuration information of the GPIO peripheral
RETURN VALUE
NONE
USAGE
static void MX_GPIO_Init(void){
/*STEP 2*/
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_RESET);
GPIO_InitStruct.Pin = led_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(led_GPIO_Port, &GPIO_InitStruct);
}
FUNCTION NAME
- GPIO_PinState HAL_GPIO_ReadPin (GPIO_TypeDef * GPIOx, uint16_t GPIO_Pin)
FUNCTION DESCRIPTION
This API is used to Read the value from a specified pin and it returns value of the type GPIO_PinState which is an enumeration in which GPIO_PIN _RESET has been assigned the value 0U and anything apart from this is GPIO_PIN_SET. This API takes in 2 arguments as input that are pointer to structure GPIO_TypeDef* GPIOx this is usually used to specify the port (A,B etc) and uint16_t GPIO_Pin this specifies the pin to be chosen and takes value from 0-15.
PARAMETERS (ARGUMENTS)
GPIOx:specifies the PORT ie X(A,B,C)
GPIO_Pin:specifies the pin or the port bit to read from i.e from 0-15.
RETURN VALUE
The input pin state value
USAGE
uint8_t buttonval = 0;
while (1)
{
buttonval=HAL_GPIO_ReadPin(BTN_GPIO_Port, BTN_PIN);
}
FUNCTION NAME
void HAL_GPIO_WritePin (GPIO_TypeDef * GPIOx, uint16_t GPIO_Pin, GPIO_PinState PinState)
FUNCTION DESCRIPTION
This API is used to write either a HIGH or LOW at the specified pin . It takes in 3 arguments as input that are pointer to structure GPIO_TypeDef* GPIOx this is usually used to specify the port (A,B etc) , uint16_t GPIO_Pin this specifies the pin to be chosen and takes value from 0-15 and GPIO_PinState PinState which is an enum of the type GPIO_PinState which takes in value SET or RESET.
PARAMETERS (ARGUMENTS)
GPIOx:specifies the PORT ie X(A,B,C)
GPIO_Pin:specifies the pin or the port bit to read from i.e from 0-15.
PinState:specifies the state to be written on the specified pin.These values are:
SET- To write a HIGH on the pin
RESET- To the clear or write LOW on the pin
RETURN VALUE
NONE
USAGE
while (1)
{
/* USER CODE END WHILE */
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_SET);
}
FUNCTION NAME
void HAL_GPIO_TogglePin (GPIO_TypeDef * GPIOx, uint16_t GPIO_Pin)
FUNCTION DESCRIPTION
This API is used to toggle or switch the state of the specified pin high to low or low to high. This API also takes in 2 arguments as input that are pointer to structure GPIO_TypeDef* GPIOx this is usually used to specify the port (A,B etc) and uint16_t GPIO_Pin this specifies the pin to be chosen and takes value from 0-15.
PARAMETERS (ARGUMENTS)
GPIOx:specifies the PORT ie X(A,B,C)
GPIO_Pin:specifies the pin or the port bit to read from i.e from 0-15.
RETURN VALUE
NONE
USAGE
while (1)
{
/* USER CODE END WHILE */
HAL_GPIO_TogglePin(led_GPIO_Port, led_Pin, GPIO_PIN_SET);
}
Data Types Involved
- uint8_t
- uint16_t
- uint32_t
- GPIO_InitTypeDef
- GPIO_PinState
- uint8-t– This data type is used to store unsigned value of 8 bits which means that only positive or zero value can be stored in the data type .Ranging from 0-255. This data type can be used to store state of a pin in a variable
- uint16_t– This data type stores unsinged values of 16 bits . The range in this case is 0-65535.This data type can be used to define pins of a port as there are 16 pins in port A
- uint32_t-This data types stores unsigned values of 32 bits i.e it stores non-negative values in the range 0 to 4,294,967,295. This data type stores 4 bytes per element.
- GPIO_InitTypeDef- A structure that helps configure pin state such as:-
- PIN-It defines the pin to be selected
- MODE-It defines the mode the specified pin has to be set in such as input , output , alternate function and even in these modes push pull , open drain etc
- PULL- It sets the pin in 3 modes which are nopull , pull up , pull down
- SPEED- It defines the speed or frequency of the operation which can be low, medium , high
5.GPIO_Pinstate- An enumerator that stores the pin state which can be SET or RESET
DEMO EXERCISE
1 CODE FOR BLINKING THE ON BOARD LED PC13 WITH A DELAY OF 1s
MX_GPIO_Init();/*STEP 1*/
while (1)
{
/* USER CODE END WHILE */
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_SET);/ *STEP 6*/
HAL_Delay(1000);/*STEP 7*/
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_RESET); /*STEP 8*/
HAL_Delay(1000);
/* USER CODE BEGIN 3 */
}
static void MX_GPIO_Init(void){
_HAL_RCC_GPIOC_CLK_ENABLE(); /*STEP 2*/
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_RESET); /*STEP 3*/
GPIO_InitStruct.Pin = led_Pin; /*STEP 4*/
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(led_GPIO_Port, &GPIO_InitStruct); /*STEP 5*/
STEPS INVOLVED
STEP 1
Create a function to configure all the pins involved in the application . Configuration such as clock enable , pin state initially, Pin no , mode, pull, speed. This function also involves HAL_GPIO_Init function that initializes the pin and has the information of its configurations.
STEP 2
Enable clock to the specified port in this case PORTC
STEP 3
Specify the initial state of the pin
STEP 4
Specify the pin no ( used a label for the pin led_Pin) the original pin no is 13 in this case. Also configure the pin mode which takes value output push pull or output open drain. Next configure the pull method which takes value no pull up and no pull down in our case.Configure the speed of the peripheral according to requirement in this case we are choosing low frequency .
STEP 5
Create the function specifying the port and all the information about the pin configuration
STEP 6
Write value to the pin as SET or HIGH
STEP 7
Include a delay in our case it is 1000ms or 1s using HAL_Delay() function
STEP 8
Write value to the pin as RESET or LOW . Since all this is in a while (1) loop the led will continue to blink
NOTE-The above exercise can also be performed by replacing the 2 HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_SET), HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_RESET) with the HAL_GPIO_TogglePin(led_GPIO_Port, led_Pin) and since the HAL API is called in the while loop the led will blink forever in a loop.
2 TURNING THE LED ON OR OFF USING A PUSH BUTTON
MX_GPIO_Init();/*STEP 1*/
uint8_t buttonval = 0;
while (1)
{
buttonval=HAL_GPIO_ReadPin(BTN_GPIO_Port, BTN_PIN);/ *STEP 7*/
/* USER CODE END WHILE */
if(buttonval == 0){
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_SET);/ *STEP 8*/
}
else{
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_RESET);/ *STEP 9*/
}
/* USER CODE BEGIN 3 */
}
static void MX_GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
/* GPIO Ports Clock Enable */
__HAL_RCC_GPIOC_CLK_ENABLE();/*STEP 2*/
__HAL_RCC_GPIOA_CLK_ENABLE();
/*Configure GPIO pin Output Level */
HAL_GPIO_WritePin(led_GPIO_Port, led_Pin, GPIO_PIN_RESET);/*STEP 3*/
/*Configure GPIO pin : led_Pin */
GPIO_InitStruct.Pin = led_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(led_GPIO_Port, &GPIO_InitStruct);/*STEP 5*/
/*Configure GPIO pin : BTN_Pin */
GPIO_InitStruct.Pin = BTN_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_NOPULL;
HAL_GPIO_Init(BTN_GPIO_Port, &GPIO_InitStruct);/*STEP 6*/
}
STEPS INVOLVED
STEP 1
Create a function to configure all the pins involved in the application . Configuration such as clock enable , pin state initially, Pin no , mode, pull, speed. This function also involves HAL_GPIO_Init function that initializes the pin and has the information of its configurations
STEP 2
Enable clock to the specified port in this case PORTC
STEP 3
Specify the initial state of the pin
STEP 4
Specify the pin no ( used a label for the pin led_Pin) the original pin no is 13 in this case. Also configure the pin mode which takes value output push pull or output open drain. Next configure the pull method which takes value no pull up and no pull down in our case.Configure the speed of the peripheral according to requirement in this case we are choosing low frequency .
STEP 5
Create the function specifying the port and all the information about the pin configuration(PORT C PIN 13)
STEP 6
Create the function specifying the port and all the information about the pin configuration(PORT A ,PIN 0)
STEP 7
Initialize a variable buttonval to read the state of the Pin PA0 using HAL api GPIO_ReadPin
STEP 8
Write value to the pin as SET or HIGH when the pin is pressed
STEP 9
Write value to the pin as RESET or LOW when the button is not pressed . Since all this is in a while (1) loop the led will continue to blink
Author

Kunal Gupta
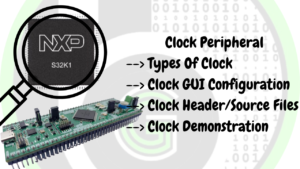

Clock Peripheral in S32K144 MCU
Clock Peripheral in S32K1 Microcontrollers. How to configure Clock in S32K1 Microcontroller. Different Clocks in S32K1 MCU’s
Using a function in Embedded C.
In this example, we will make animations by turning off and on Leds with some delays. We could simply do it like this. We could simply do it like this. PORTB=0B10101010; _delay_ms(1000); PORTB=0B01010101; _delay_ms(1000); . . . PORTB=0B01000001; _delay_ms(1000); But instead of writing like this, we would use the function Led_pin( ). It will reduce the typing a bit but in the next blog, we will learn to make it very small by learning a concept called Bit Shifting. Let\’s go for an example. #define F_CPU 16000000UL //defining the clock speed of the processor #include <avr/io.h> // library for using registers [PORTX,PORTD,PINX] #include <util/delay.h> // library for using delay void Led_pin(uint8_t byte) // Making a function with argument \”byte\” having data type unsigned int 8. { PORTB = byte; // Whenever the function is called it will store the argument byte in PORTB with delay. _delay_ms(100); } int main(void) { DDRB = (0b00111110); while(1) { Led_pin(0b00101010); Led_pin(0b00010100); Led_pin(0b00101110); Led_pin(0b00010001); Led_pin(0b00101010); Led_pin(0b00011100); Led_pin(0b00101011); Led_pin(0b00010100); PORTB=0b00000000; _delay_ms(1000); } return(0); } In the above code we made a function Led_pin( ). void Led_pin(uint8_t byte) { PORTB = byte; _delay_ms(100); } Here Led_pin takes a single byte of data type unsigned int [uint8_t]. Whenever our code calls this function the arguments inside it directly go to the PORTB and blink for 100 microseconds asset in delay. OUTPUT If you are new and feeling confused about other libraries and functions then please refer to this blog. Author: Kunal Gupta
S32K1xx Automotive MCU course( Intermediate)
DAC PDB MTB(Micro Trace Buffer) Miscellaneous Control Module(MCM) System Integration Module Crossbar Switch Lite Memory Protection Unit Peripheral Bridge Trigger MUX Control External watchdog Monitor Error Injection Module Error reporting module CRC Watchdog timer DAC Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo. PDB Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo. MTB(Micro Trace Buffer) Tab Content Miscellaneous Control Module(MCM) Tab Content System Integration Module Tab Content Crossbar Switch Lite Tab Content Memory Protection Unit Tab Content Peripheral Bridge Tab Content Trigger MUX Control Tab Content External watchdog Monitor Tab Content Error Injection Module Tab Content Error reporting module Tab Content CRC Tab Content Watchdog timer Tab Content CAN FlexIO LIN CAN Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo. FlexIO Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo. LIN Tab Content Author: Kunal Gupta
Introduction to STM32WB55
Table of Contents About STMicroelectronics STMicroelectronics is a leading provider of semiconductor solutions that are seamlessly integrated into billions of electronic devices used by people worldwide on a daily basis. The semiconductor company builds products, solutions, and ecosystems that enable smarter mobility, more efficient power and energy management, and the wide-scale deployment of the Internet of Things and connectivity technologies. To know more about STMicroelectronics refer to its website: www.st.com. Going back in history, ST was formed in 1987 by the merger of two government-owned semiconductor companies: Italian SGS Microelettronica (where SGS stands for Società Generale Semiconduttori, “Semiconductors’ General Company”), and French Thomson Semiconductors, the semiconductor arm of Thomson. In this blog, we are going to start with ST IoT-based Nucleo Board STm32WB55. What is STM32WB Series all about? The STM32WB55xx and STM32WB35xx are advanced multiprotocol wireless devices that boast ultra-low-power consumption. These devices are equipped with a powerful and efficient radio that is compliant with the Bluetooth® Low Energy SIG specification 5 and IEEE 802.15.4-2011 (Zigbee). Additionally, they feature a dedicated Arm® Cortex®-M0+ processor that handles all real-time low-layer operations. These cutting-edge devices are perfect for a wide range of applications that require reliable and efficient wireless communication. Whether you’re working on a smart home project, a wearable device, or an industrial automation system, the STM32WB55xx and STM32WB35xx are the ideal choices. With their advanced features and capabilities, these devices are sure to revolutionize the way we think about wireless communication. So why wait? Start exploring the possibilities today and discover what the STM32WB55xx and STM32WB35xx can do for you! The devices have been meticulously crafted to operate on minimal power and are built around the high-performance Arm® Cortex®-M4 32-bit RISC core, which can operate at a frequency of up to 64 MHz. This core boasts a Floating-point unit (FPU) single precision that supports all Arm® single-precision data-processing instructions and data types. Additionally, it is equipped with a full set of DSP instructions and a memory protection unit (MPU) that enhances application security. These devices have been designed with the utmost care and attention to detail, ensuring that they are not only efficient but also highly effective. The Arm® Cortex®-M4 32-bit RISC core is a powerful tool that enables these devices to perform at an exceptional level, while the FPU single precision and DSP instructions provide unparalleled accuracy and precision. Furthermore, the memory protection unit (MPU) ensures that your applications are secure and protected from any potential threats. Enhanced inter-processor communication is provided by the IPCC with six bidirectional channels. The HSEM provides hardware semaphores used to share common resources between the two processors. The devices embed high-speed memories (up to 1 Mbyte of flash memory for STM32WB55xx, up to 512 Kbytes for STM32WB35xx, up to 256 Kbytes of SRAM for STM32WB55xx, 96 Kbytes for STM32WB35xx), a Quad-SPI flash memory interface (available on all packages) and an extensive range of enhanced I/Os and peripherals.  About STM32WB55 Architecture Memories Security and Safety True random number generator (RNG) RF Subsystem Low Power Modes Clocks and Startup General Purpose Input Output(GPIOs) Direct Memory Access (DMA) Interrupts and Events Analog to Digital Convertor (ADC) Comparators (COMP) Touch Sensing Controller Liquid crystal display controller (LCD) Timers and watchdogs Real-time clock (RTC) and backup registers Inter Integrated Circuit (I2C) Universal Synchronous/Asynchronous Receiver Transmitter (USART) Serial Peripheral Interface(SPI) Serial audio interfaces (SAI) Quad-SPI memory interface (QUADSPI) Architecture Architecture STM32WB55 Architecture The host application is housed on an Arm® Cortex®-M4 CPU (named CPU1) that connects with a generic microcontroller subsystem. The RF subsystem is made up of a specialized Arm® Cortex®-M0+ microprocessor (named CPU2), Bluetooth Low Energy and 802.15.4 digital MAC blocks, an RF analog front end, and proprietary peripherals. All Bluetooth Low Energy and 802.15.4 low-layer stack functions are handled by the RF subsystem, which limits communication with the CPU1 to high-level exchanges. Some functions are shared between the RF subsystem CPU (CPU2) and the Host CPU (CPU1): Flash memories SRAM1, SRAM2a, and SRAM2b (SRAM2a can be retained in Standby mode) Security peripherals (RNG, AES1, PKA) Clock RCC Power control (PWR) Memories Memories STM32WB55 Memories 2.1. Adaptive real-time memory accelerator (ART Accelerator) The ART Accelerator is a memory accelerator optimized for STM32 industry-standard Arm® Cortex®-M4 processors. It balances the inherent performance advantage of the Arm® Cortex®-M4 over flash memory technologies. To release the processor near 80 DMIPS performance at 64 MHz, the accelerator implements an instruction prefetch queue and branch cache, which increases program execution speed from the 64-bit flash memory. Based on CoreMark benchmark, the performance achieved thanks to the ART accelerator is equivalent to 0 wait state program execution from flash memory at a CPU frequency up to 64 MHz. 2.2. Memory protection unit In order to prevent one task from unintentionally corrupting the memory or resources used by any other active task, the memory protection unit (MPU) is used to manage the CPU1’s accesses to memory. This memory area is organized into up to eight protected areas. The MPU is especially helpful for applications where some critical or certified code must be protected against the misbehavior of other tasks. It is usually managed by an RTOS (real-time operating system). 2.3. Embedded flash memory The STM32WB55xx and STM32WB35xx devices feature, respectively, up to 1 Mbyte and 512 Kbytes of embedded flash memory available for storing programs and data, as well as some customer keys. 2.4. Embedded SRAM The STM32WB55xx devices feature up to 256 Kbytes of embedded SRAM, split in three blocks: SRAM1: up to 192 Kbytes mapped at address 0x2000 0000 SRAM2a: 32 Kbytes located at address 0x2003 0000 also mirrored at 0x1000 0000, with hardware parity check (this SRAM can be retained in Standby mode) SRAM2b: 32 Kbytes located at address 0x2003 8000 (contiguous with SRAM2a) and mirrored at 0x1000 8000 with hardware parity check. Security and Safety Security and Safety The STM32WB55xx contain many security blocks both for the Bluetooth Low Energy or IEEE 802.15.4 and the Host application. It includes: Customer storage of the Bluetooth Low Energy and
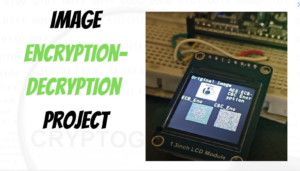

Cryptography Do It Yourself (DIY) projects
Learn Cryptography technology in embedded through DIY projects using NXP S32K144 Automotive Chips
SPI Peripheral in S32K144
Explore this blog to know about SPI peripheral in NXP S32K144 MCU

Author: Kunal Gupta

Author